Buton + Modal ile yapılmış başvuru sistemidir
Onay veya red verip dm yoluyla geri dönüş yamaktadır
DAHA ÇOK BÖYLE KOMUTLAR VEYA BOTLAR İSTİYORSANIZ TEPKI BIRAKABILIRSINIZ
Onay veya red verip dm yoluyla geri dönüş yamaktadır
DAHA ÇOK BÖYLE KOMUTLAR VEYA BOTLAR İSTİYORSANIZ TEPKI BIRAKABILIRSINIZ
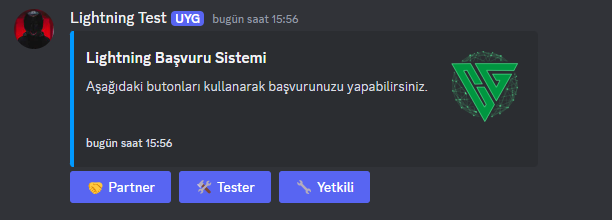
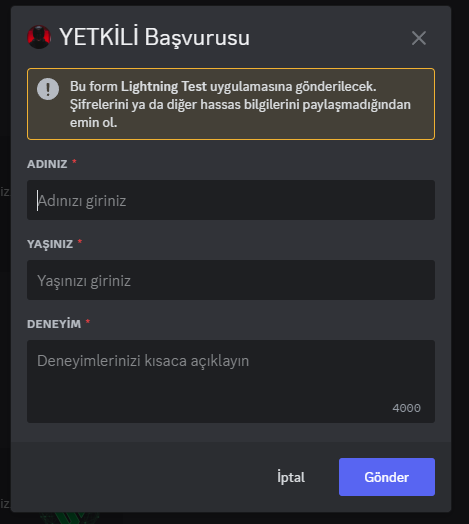
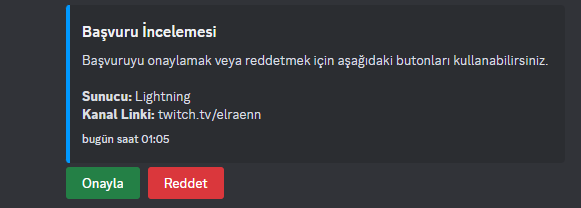
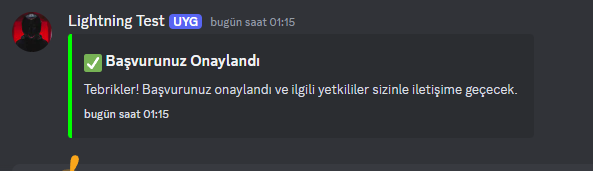
JavaScript:
const { ActionRowBuilder, ButtonBuilder, ButtonStyle, EmbedBuilder, ModalBuilder, TextInputBuilder, TextInputStyle } = require('discord.js');
exports.run = async (client, message, args) => {
// Embed Mesajı
const embed = new EmbedBuilder()
.setColor('#0099ff')
.setTitle('Lightning Başvuru Sistemi')
.setDescription('Aşağıdaki butonları kullanarak başvurunuzu yapabilirsiniz.')
.setThumbnail('Mesajın Sağ Tarafına Eklenen Küçük Fotoğraf') // BURAYI DOLDUR
// Butonlar
const buttons = new ActionRowBuilder()
.addComponents(
new ButtonBuilder()
.setCustomId('apply_partner')
.setLabel('🤝 Partner')
.setStyle(ButtonStyle.Primary),
new ButtonBuilder()
.setCustomId('apply_tester')
.setLabel('🛠️ Tester')
.setStyle(ButtonStyle.Primary),
new ButtonBuilder()
.setCustomId('apply_staff')
.setLabel('🔧 Yetkili')
.setStyle(ButtonStyle.Primary),
);
// Mesajı gönder
await message.channel.send({ embeds: [embed], components: [buttons] });
// Buton etkileşimleri
const filter = (interaction) => interaction.isButton();
const collector = message.channel.createMessageComponentCollector({ filter, time: 60000 });
collector.on('collect', async (interaction) => {
let modal;
if (interaction.customId === 'apply_partner' || interaction.customId === 'apply_tester') {
// Partner veya Tester başvurusu için modal oluştur
modal = new ModalBuilder()
.setCustomId('applicationModal')
.setTitle(`${interaction.customId.replace('apply_', '').toUpperCase()} Başvurusu`);
const serverInput = new TextInputBuilder()
.setCustomId('serverInput')
.setLabel('Sunucu Adınız')
.setStyle(TextInputStyle.Short)
.setPlaceholder('Sunucu adınızı giriniz')
.setRequired(true);
const channelLinkInput = new TextInputBuilder()
.setCustomId('channelLinkInput')
.setLabel('Deneyiminiz')
.setStyle(TextInputStyle.Short)
.setPlaceholder('Deneyiminizi Yazın')
.setRequired(true);
const row1 = new ActionRowBuilder().addComponents(serverInput);
const row2 = new ActionRowBuilder().addComponents(channelLinkInput);
modal.addComponents(row1, row2);
} else if (interaction.customId === 'apply_staff') {
// Yetkili başvurusu için modal oluştur
modal = new ModalBuilder()
.setCustomId('staffApplicationModal')
.setTitle('YETKİLİ Başvurusu');
const nameInput = new TextInputBuilder()
.setCustomId('nameInput')
.setLabel('Adınız')
.setStyle(TextInputStyle.Short)
.setPlaceholder('Adınızı giriniz')
.setRequired(true);
const ageInput = new TextInputBuilder()
.setCustomId('ageInput')
.setLabel('Yaşınız')
.setStyle(TextInputStyle.Short)
.setPlaceholder('Yaşınızı giriniz')
.setRequired(true);
const experienceInput = new TextInputBuilder()
.setCustomId('experienceInput')
.setLabel('Deneyim')
.setStyle(TextInputStyle.Paragraph)
.setPlaceholder('Deneyimlerinizi kısaca açıklayın')
.setRequired(true);
const row1 = new ActionRowBuilder().addComponents(nameInput);
const row2 = new ActionRowBuilder().addComponents(ageInput);
const row3 = new ActionRowBuilder().addComponents(experienceInput);
modal.addComponents(row1, row2, row3);
}
await interaction.showModal(modal);
});
// Modal Etkileşimleri
client.on('interactionCreate', async (interaction) => {
if (interaction.isModalSubmit()) {
let logDescription = '';
if (interaction.customId === 'applicationModal') {
const serverName = interaction.fields.getTextInputValue('serverInput');
const channelLink = interaction.fields.getTextInputValue('channelLinkInput');
logDescription = `**Sunucu:** ${serverName}\n**Kanal Linki:** ${channelLink}`;
} else if (interaction.customId === 'staffApplicationModal') {
const name = interaction.fields.getTextInputValue('nameInput');
const age = interaction.fields.getTextInputValue('ageInput');
const experience = interaction.fields.getTextInputValue('experienceInput');
logDescription = `**Adı:** ${name}\n**Yaş:** ${age}\n**Deneyim:** ${experience}`;
}
// Kullanıcıya "Başvurunuz Alınmıştır." mesajı gönder
await interaction.reply({ content: '**Başvurunuz Alınmıştır.**', ephemeral: true });
// Onay/Red Embed Mesajı
const approvalEmbed = new EmbedBuilder()
.setColor('#0099ff')
.setTitle('Başvuru İncelemesi')
.setDescription(`Başvuruyu onaylamak veya reddetmek için aşağıdaki butonları kullanabilirsiniz.\n\n${logDescription}`)
.setTimestamp();
const approvalButtons = new ActionRowBuilder()
.addComponents(
new ButtonBuilder()
.setCustomId('approve')
.setLabel('Onayla')
.setStyle(ButtonStyle.Success),
new ButtonBuilder()
.setCustomId('reject')
.setLabel('Reddet')
.setStyle(ButtonStyle.Danger),
);
const logChannel = client.channels.cache.get('BURAYA_LOG_KANAL_ID_GIR'); // Log kanalı ID'si buraya yazılmalı
if (logChannel) {
await logChannel.send({ embeds: [approvalEmbed], components: [approvalButtons] });
}
}
// Onay/Red Buton Etkileşimleri
if (interaction.isButton()) {
const applicant = interaction.user;
if (interaction.customId === 'approve') {
const approvedEmbed = new EmbedBuilder()
.setColor('#00FF00')
.setTitle('✅ Başvurunuz Onaylandı')
.setDescription('Tebrikler! Başvurunuz onaylandı ve ilgili yetkililer sizinle iletişime geçecek.')
.setTimestamp();
// Başvuru sahibine DM olarak gönder
await applicant.send({ embeds: [approvedEmbed] });
// Log kanalındaki mesajı güncelle
await interaction.update({ content: 'Başvuru onaylandı.', components: [] });
} else if (interaction.customId === 'reject') {
const rejectedEmbed = new EmbedBuilder()
.setColor('#FF0000')
.setTitle('❌ Başvurunuz Reddedildi')
.setDescription('Üzgünüz, başvurunuz reddedildi. Başka bir zaman tekrar deneyebilirsiniz.')
.setTimestamp();
// Başvuru sahibine DM olarak gönder
await applicant.send({ embeds: [rejectedEmbed] });
// Log kanalındaki mesajı güncelle
await interaction.update({ content: 'Başvuru reddedildi.', components: [] });
}
}
});
};
exports.conf = {
aliases: []
};
exports.help = {
name: "basvurubutonu"
};